Section 2.2 C Pointer Variables
Checkpoint 2.2.2. Using Pointers.
- When you want to hold a normal int
- When you want to dynamically allocate memory
- When you want to make a fixed-size array of size 10
- When you want to make a linked dynamic data structure
- When you want to pass a large data structure to a function
- When you want to pass a parameter to a function that that function can change
- When you want to hold a floating point number
Which of these are good reasons to use a pointer variable? Select all that are true.
Hint.
What is the unique feature of using pointer instead of value? Do we need these features in the scenarios above?
Checkpoint 2.2.3. Reading/Tracing Code with Pointers.
Given the following code, what does it print to the screen when executed?
int a, *ptr;
a = 3;
ptr = &a;
*ptr = 7;
printf("%d\n", *ptr);
//draw what memory looks like here.
Trace through its execution and draw a picture of memory after its execution.
Answer.
Here is a picture showing the result of the above pointer operatons. Variable
a
’s initial value is 3, while ptr
contains the address of a
. Dereferencing ptr
and setting its associated value to 7 thus updates the value of a
to 7.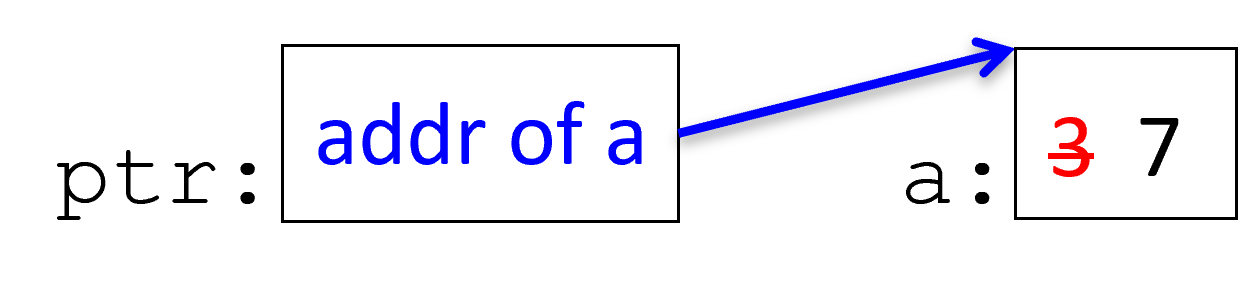
Checkpoint 2.2.4. Reading/Tracing Code with Pointers.
- Segfault / Crash (Probably)
- 10
- 20
- 30
- 0
What does the following code print to the screen when executed?
int x, y, *ptr, z;
ptr = NULL;
x = 10;
y = 20;
z = 30;
printf("%d\n", *ptr);
ptr = &x;
Hint.
What happens when you dereference a NULL pointer?
Checkpoint 2.2.5. Reading/Tracing Code with Pointers.
What does the following code print to the screen when executed?
int a, b, *ptr2;
a = 5;
b = 6;
ptr2 = &b;
b = 10;
printf("%d\n", *ptr2);
Checkpoint 2.2.6. Reading/Tracing Code with Pointers.
- *ptr1=10 *ptr2=6
- Correct! Good job!
- *ptr1=&x *ptr2=&y
- The printf statement dereferences the values.
- *ptr1=2 *ptr2=10
- Emm...please think about it again.
- *ptr1=6 *ptr2=10
- Emm...please think about it again.
- *ptr1=2 *ptr2=2
- Emm...please think about it again.
- *ptr1=10 *ptr2=10
- Emm...please think about it again.
- *ptr1=6 *ptr2=6
- Emm...please think about it again.
- segfault (program crashes with a segfault)
- This code actually produces output!
- compile error
- This code actually compiles!
Given the following code:
void f1(void) {
int x, y, *ptr1, *ptr2;
x = 2;
y = 10;
ptr1 = &x;
ptr2 = ptr1;
*ptr1 = 6;
ptr1 = &y;
printf("*ptr1=%d *ptr2=%d\n", *ptr1, *ptr2);
}
What does the function f1() print?
Hint.
Trace the values in memory for the variables with arrows and boxes.
Checkpoint 2.2.7. Swapping Values with Pointers.
Reorder the lines of code below to make a program that swaps the values pointed to by the integer pointers ptr1 and ptr2 (int *ptr1, *ptr2). There is also an integer x (int x).
Assume the follwoing variable declaration and initialized code:
int x, y, temp;
int *ptr1, *ptr2;
x = 6;
y = 7;
ptr1 = &x;
ptr2 = &y;